Create KDS Order Over Local Network
Overview
Once you’ve established a connection to a KDS screen you can create KDS orders using TCP. If successful Fresh KDS will return a parsed integration id.
Body Parameters
Property |
Required |
Type |
Description |
command |
yes |
string |
accepted values: create-order , update-order , cancel-order |
order |
yes |
Order |
object containing KDS order data |
command
property added in version 2.21.30, for backwards compatibility default value is create-order
Order
Property |
Required |
Type |
Description |
id |
yes |
string |
unique id of this KDS order |
name |
yes |
string |
order/customer name |
time |
yes |
int |
milliseconds since epoch |
pickupTime |
no |
date |
date/time for order pickup in ISO 8601 format |
phoneNumber |
no |
string |
customer phone number in E.164 format |
optInForSms |
no |
bool |
if false KDS will not message customer for this order; default is true |
deliveryAddress |
no |
string |
delivery address for order |
mode |
yes |
string |
accepted values: For Here, ToGo, Pickup, DriveThru, Delivery, Curbside |
deliveryHandoff |
no |
bool |
if mode is Delivery and this is true order will display as Delivery Handoff |
server |
no |
string |
name of employee creating the order |
items |
yes |
KdsItem[] |
array of menu items |
terminal |
no |
string |
POS terminal creating the order |
specialInstructions |
no |
string |
special instructions for the order |
customerArrivedUrl |
no |
url |
if present the URL will be added to the order received and order ready messages |
vehicleModel |
no |
string |
vehicle make and model |
vehicleColor |
no |
string |
vehicle color |
costs |
no |
Costs |
object with order costs |
deliveryService |
no |
DeliveryService |
object with delivery service information |
originSource |
no |
string |
optional parameter that gets appended to the order name |
checkNumber |
no |
string |
optional parameter that gets appended to the order name |
priority |
no |
bool |
if true order card will be flagged as priority and moved to front of queue |
KDS Item
Property |
Required |
Type |
Description |
id |
no |
string |
id of item |
lineId |
no |
string |
must be unique within the order; used for processing order update command |
name |
yes |
string |
name of item |
qty |
yes |
int |
quantity of item |
price |
no |
string |
price of item; can include currency symbol |
mods |
yes |
Modifier[] |
array of item modifiers; send empty array for items without modifiers |
specialInstructions |
no |
string |
special instructions for item |
course |
no |
string |
course name/number of the item |
courseStatus |
no |
string |
valid values are “straightFire”, “hold”, “fire”, and “pickup” |
seat |
no |
string |
seat number of the item |
Modifier
Property |
Required |
Type |
Description |
id |
no |
string |
id of modifier |
name |
yes |
string |
name of modifier |
previously item.mods
was a string[], which is still supported for backwards compatibility
Costs
Property |
Required |
Type |
Description |
subtotal |
no |
string |
subtotal of order |
tax |
no |
string |
tax on order |
deliveryFee |
no |
string |
delivery fee on order |
surcharge |
no |
string |
surcharge on order |
convenienceFee |
no |
string |
convenience fee on order |
tip |
no |
string |
tip on order |
additionalFees |
no |
additionalFees[] |
array of additional fees |
total |
no |
string |
total of order |
promoCodes |
no |
promoCodes[] |
array of promo codes on order |
all properties in the Costs object are strings and can include currency symbols for display
Additional Fees
Property |
Required |
Type |
Description |
name |
yes |
string |
name of fee |
amount |
yes |
string |
amount of fee; can include currency symbol |
Property |
Required |
Type |
Description |
name |
no |
string |
name of promo code |
amount |
yes |
string |
amount of promo; can include currency symbol |
Delivery Service
Property |
Required |
Type |
Description |
name |
yes |
string |
name of delivery service |
orderId |
yes |
string |
delivery service order ID |
driverPhone |
no |
string |
delivery driver phone number in E.164 format |
Example
{
"command": "create-order",
"order": {
"id": "123",
"name": "John Doe",
"time": 1551454803184,
"pickupTime": "2023-04-03T14:15:00.000Z",
"phoneNumber": "+16155551234",
"mode": "Pickup",
"items": [{
"lineId": "1",
"name": "Hamburger",
"qty": 1,
"mods": ["No Ketchup", "Side Fries"],
"course": "Course 1",
"seat": "Guest 1",
"courseStatus": "fire"
},
{
"lineId": "2",
"name": "Vanilla Shake",
"qty": 1,
"mods": [],
"course": "Course 2",
"seat": "Guest 1",
"courseStatus": "hold"
}
]
}
}
Examples of Object Mapping to KDS Screens
KDS Order
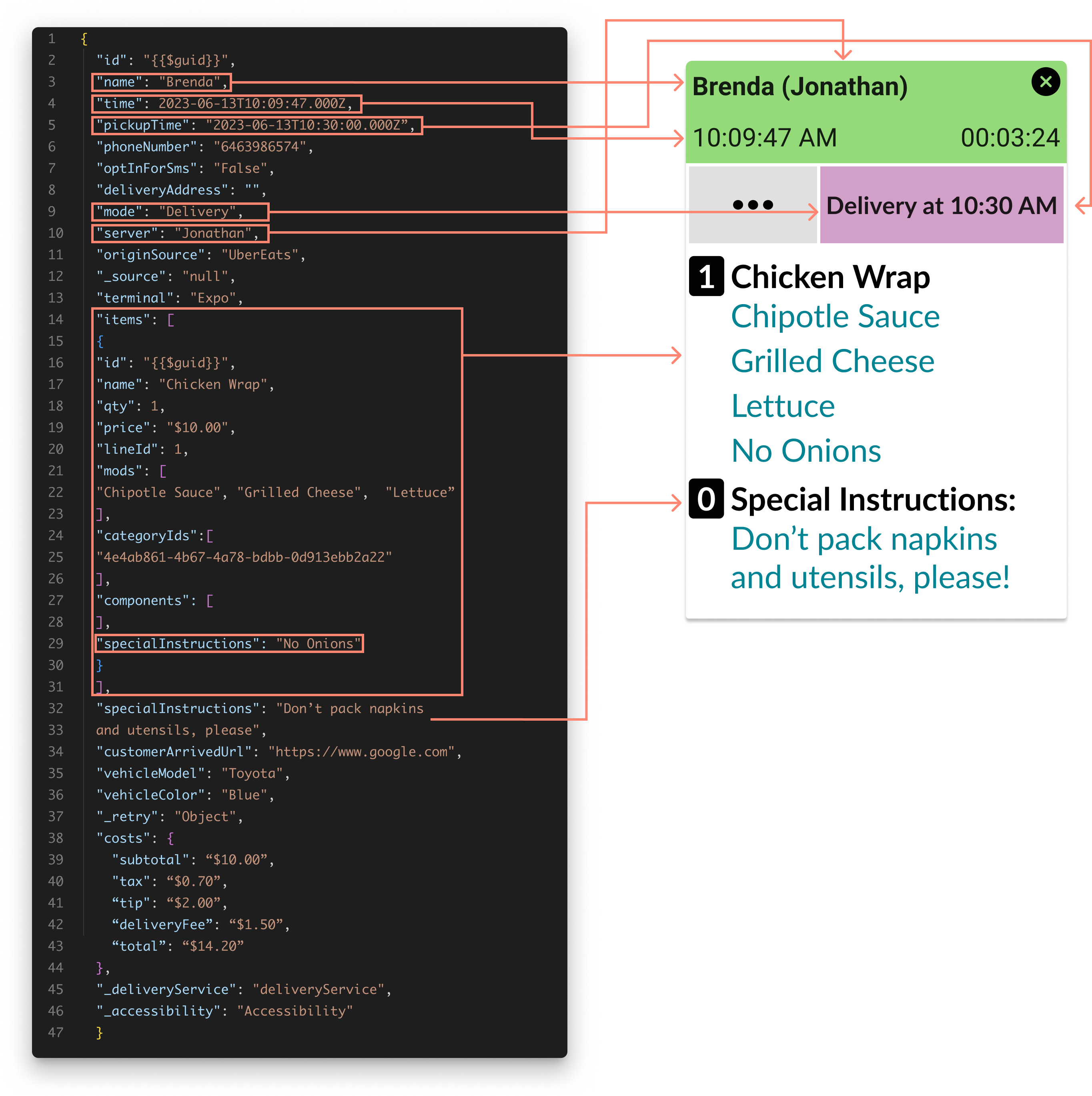
Take Out Order - Delivery
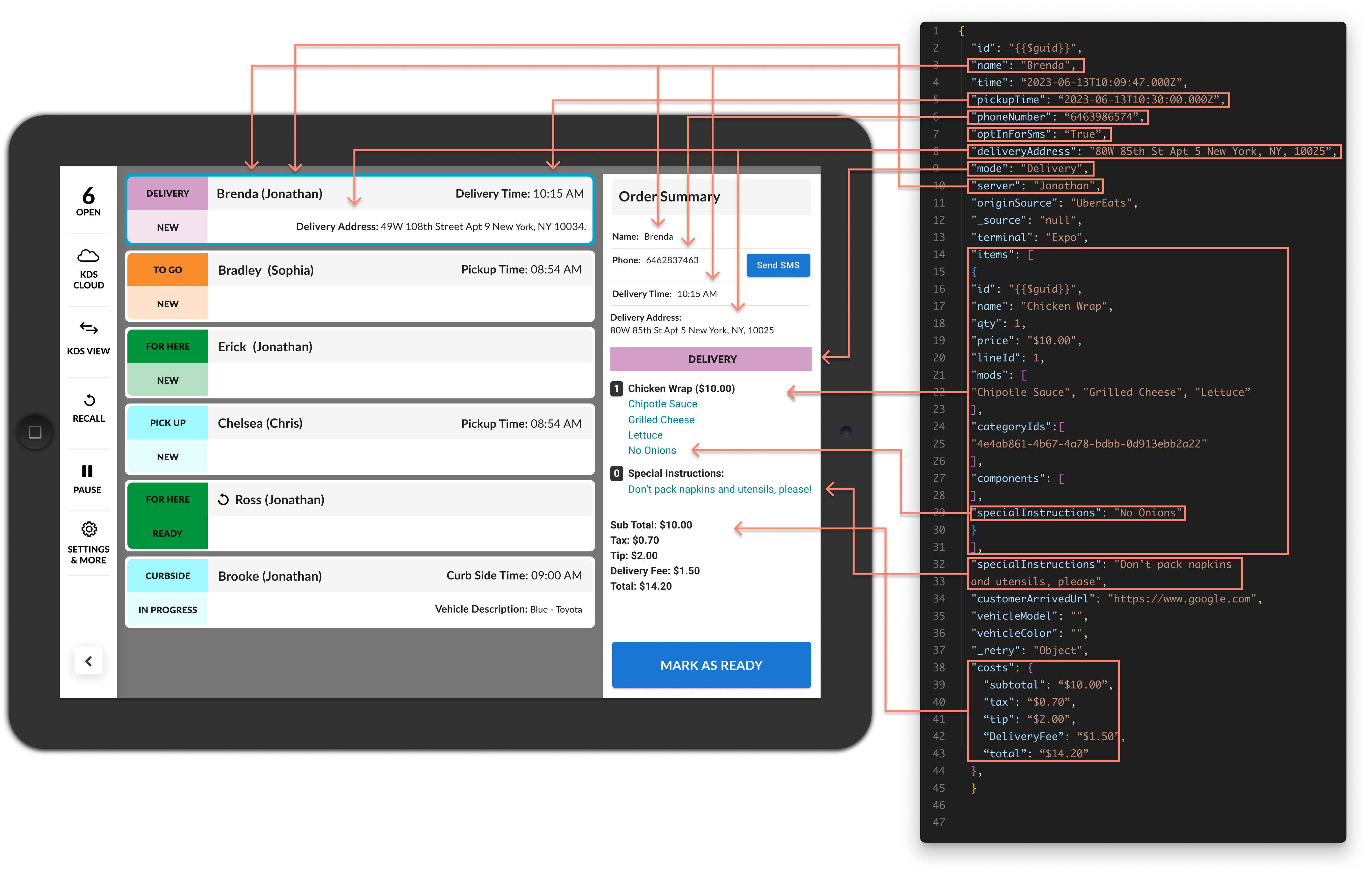
Take Out Order - Curbside
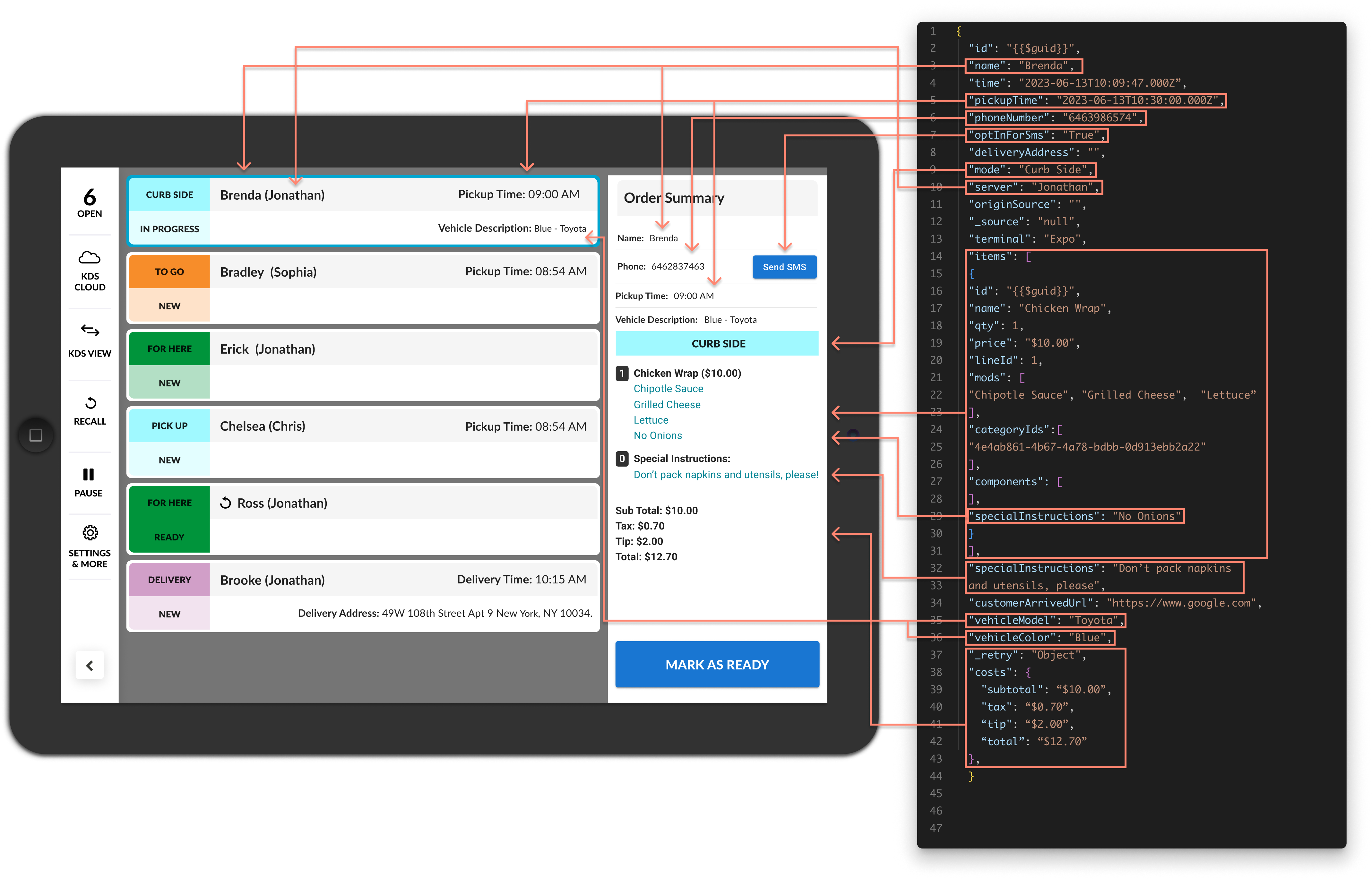
Responses
Response Type |
Response Value |
Success |
order.id value |
Failure |
Unable to parse data |
Failure |
DUPLICATE_ORDER_ID - An order with the same order.id is on the screen or is on hold |